HandlerMethod 등록
스프링에서 requestMappingHandlerMapping
이 생성되며 선언한 컨트롤러의 메서드들이 요청처리를 위한 메타정보과 함께 HandlerMethod
로 등록된다.
public HandlerMethod(
String beanName, BeanFactory beanFactory,
@Nullable MessageSource messageSource, Method method) {
super(method);
Assert.hasText(beanName, "Bean name is required");
Assert.notNull(beanFactory, "BeanFactory is required");
this.bean = beanName;
this.beanFactory = beanFactory;
this.messageSource = messageSource;
Class<?> beanType = beanFactory.getType(beanName);
if (beanType == null) {
throw new IllegalStateException("Cannot resolve bean type for bean with name '" + beanName + "'");
}
this.beanType = ClassUtils.getUserClass(beanType);
this.validateArguments = false;
this.validateReturnValue = false;
evaluateResponseStatus();
this.description = initDescription(this.beanType, method);
}
DispatcherServlet 생성
DispatcherServlet
는 DispatcherServletAutoConfiguration
를 통해 생성된다.
@Bean(name = DEFAULT_DISPATCHER_SERVLET_BEAN_NAME)
public DispatcherServlet dispatcherServlet(WebMvcProperties webMvcProperties) {
DispatcherServlet dispatcherServlet = new DispatcherServlet();
dispatcherServlet.setDispatchOptionsRequest(webMvcProperties.isDispatchOptionsRequest());
dispatcherServlet.setDispatchTraceRequest(webMvcProperties.isDispatchTraceRequest());
configureThrowExceptionIfNoHandlerFound(webMvcProperties, dispatcherServlet);
dispatcherServlet.setPublishEvents(webMvcProperties.isPublishRequestHandledEvents());
dispatcherServlet.setEnableLoggingRequestDetails(webMvcProperties.isLogRequestDetails());
return dispatcherServlet;
}
applicationContext 주입
DispatcherServlet
는 ApplicationContextAware
를 확장하고 있기에 빈이 생성되며 ApplicationContextAwareProcessor
에 의해 applicationContext
가 DispatcherServlet
에 설정된다.
// ApplicationContextAwareProcessor
public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {
if (bean instanceof Aware) {
invokeAwareInterfaces(bean);
}
return bean;
}
private void invokeAwareInterfaces(Object bean) {
// ...
if (bean instanceof ApplicationContextAware applicationContextAware) {
applicationContextAware.setApplicationContext(this.applicationContext);
}
}
DispatcherServlet
는 아래 같은 복잡한 계층 구조를 가지고 있는데 DispatcherServlet
가 생성되는 과정에서 필요한 부분만 살펴보면 다음과 같다.
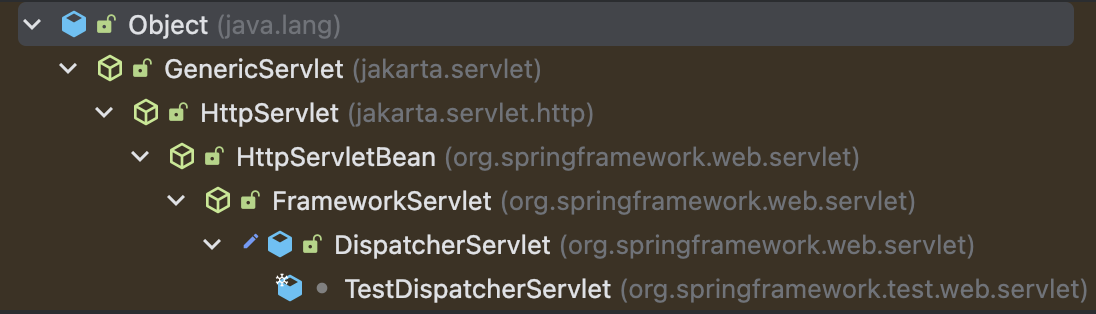
Servlet Bean 초기화
HttpServletBean
은 GenericServelt
의 init
를 재정의하고 있다.
해당 메서드 안에서는 FrameworkServlet
이 재정의한 HttpServletBean
의 initServletBean
을 실행한다.
public final void init() throws ServletException {
// ...
// Let subclasses do whatever initialization they like.
initServletBean();
}
webApplicationContext 초기화
FrameworkServlet
의 initServletBean
에서는 initWebApplicationContext
를 통해 webApplicationContext
가 초기화된다.
// FrameworkServlet
protected final void initServletBean() throws ServletException {
// ...
try {
this.webApplicationContext = initWebApplicationContext();
initFrameworkServlet();
}
catch (ServletException | RuntimeException ex) {
logger.error("Context initialization failed", ex);
throw ex;
}
// ..
}
DispatcherServlet 갱신
이후 webApplicationContext
이 DispatcherServlet
이 재정의한 FrameworkServelet
의 onRefresh
메서드를 통해 스프링에서 설정하여 빈으로 등록된 MultipartResolver
, LocaleResolver
, ThemeResolver
, HandlerMappings
, HandlerAdapters
, HandlerExceptionResolvers
, RequestToViewNameTranslator
, ViewResolvers
, FlashMapManager
가 초기화된다.
// DispatcherServlet
protected WebApplicationContext initWebApplicationContext() {
// ..
if (!this.refreshEventReceived) {
// Either the context is not a ConfigurableApplicationContext with refresh
// support or the context injected at construction time had already been // refreshed -> trigger initial onRefresh manually here. synchronized (this.onRefreshMonitor) {
onRefresh(wac);
}
}
// ..
}
protected void onRefresh(ApplicationContext context) {
initStrategies(context);
}
protected void initStrategies(ApplicationContext context) {
initMultipartResolver(context);
initLocaleResolver(context);
initThemeResolver(context);
initHandlerMappings(context);
initHandlerAdapters(context);
initHandlerExceptionResolvers(context);
initRequestToViewNameTranslator(context);
initViewResolvers(context);
initFlashMapManager(context);
}
요청 처리
위와 같은 과정을 통해 요청을 처리할 준비를 마무리하고 서버가 시작되며 실제 요청을 처리한다.DispatcherServlet
의 doService
를 통해 요청 처리가 이루어지는데 이때 HandlerMethod
를 사용하여 요청을 처리한다.
이 과정에서 HandlerMethod
는 아직 실제 빈이 주입되어 있지 않을 수 있다. 이를 위해 요청을 처리할 땐 createWithResolvedBean
에서 HandlerMethod
에 등록된 빈이 String
타입의 빈 이름이라면 실제 빈으로 교체한 이후 요청을 처리한다.
public HandlerMethod createWithResolvedBean() {
Object handler = this.bean;
if (this.bean instanceof String beanName) {
Assert.state(this.beanFactory != null, "Cannot resolve bean name without BeanFactory");
handler = this.beanFactory.getBean(beanName);
}
Assert.notNull(handler, "No handler instance");
return new HandlerMethod(this, handler, false);
}
'스프링' 카테고리의 다른 글
Webflux, ReactiveTransactionManager 환경에서의 이벤트 발행 (0) | 2025.03.24 |
---|---|
인터페이스 타입으로 등록한 빈을 구현체 타입으로 DI 받지 못한 이유 (0) | 2025.03.19 |
트랜잭션을 위한 프록시 객체와 AspectJ의 프록시 객체는 어떤 순서로 실행될까? (0) | 2025.03.18 |
내가 이해한 스프링의 빈 생명 주기 (0) | 2025.03.17 |
트랜잭션 커밋과 AOP 중 어떤 것이 먼저 실행 될까? (0) | 2025.03.15 |
HandlerMethod 등록
스프링에서 requestMappingHandlerMapping
이 생성되며 선언한 컨트롤러의 메서드들이 요청처리를 위한 메타정보과 함께 HandlerMethod
로 등록된다.
public HandlerMethod(
String beanName, BeanFactory beanFactory,
@Nullable MessageSource messageSource, Method method) {
super(method);
Assert.hasText(beanName, "Bean name is required");
Assert.notNull(beanFactory, "BeanFactory is required");
this.bean = beanName;
this.beanFactory = beanFactory;
this.messageSource = messageSource;
Class<?> beanType = beanFactory.getType(beanName);
if (beanType == null) {
throw new IllegalStateException("Cannot resolve bean type for bean with name '" + beanName + "'");
}
this.beanType = ClassUtils.getUserClass(beanType);
this.validateArguments = false;
this.validateReturnValue = false;
evaluateResponseStatus();
this.description = initDescription(this.beanType, method);
}
DispatcherServlet 생성
DispatcherServlet
는 DispatcherServletAutoConfiguration
를 통해 생성된다.
@Bean(name = DEFAULT_DISPATCHER_SERVLET_BEAN_NAME)
public DispatcherServlet dispatcherServlet(WebMvcProperties webMvcProperties) {
DispatcherServlet dispatcherServlet = new DispatcherServlet();
dispatcherServlet.setDispatchOptionsRequest(webMvcProperties.isDispatchOptionsRequest());
dispatcherServlet.setDispatchTraceRequest(webMvcProperties.isDispatchTraceRequest());
configureThrowExceptionIfNoHandlerFound(webMvcProperties, dispatcherServlet);
dispatcherServlet.setPublishEvents(webMvcProperties.isPublishRequestHandledEvents());
dispatcherServlet.setEnableLoggingRequestDetails(webMvcProperties.isLogRequestDetails());
return dispatcherServlet;
}
applicationContext 주입
DispatcherServlet
는 ApplicationContextAware
를 확장하고 있기에 빈이 생성되며 ApplicationContextAwareProcessor
에 의해 applicationContext
가 DispatcherServlet
에 설정된다.
// ApplicationContextAwareProcessor
public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {
if (bean instanceof Aware) {
invokeAwareInterfaces(bean);
}
return bean;
}
private void invokeAwareInterfaces(Object bean) {
// ...
if (bean instanceof ApplicationContextAware applicationContextAware) {
applicationContextAware.setApplicationContext(this.applicationContext);
}
}
DispatcherServlet
는 아래 같은 복잡한 계층 구조를 가지고 있는데 DispatcherServlet
가 생성되는 과정에서 필요한 부분만 살펴보면 다음과 같다.
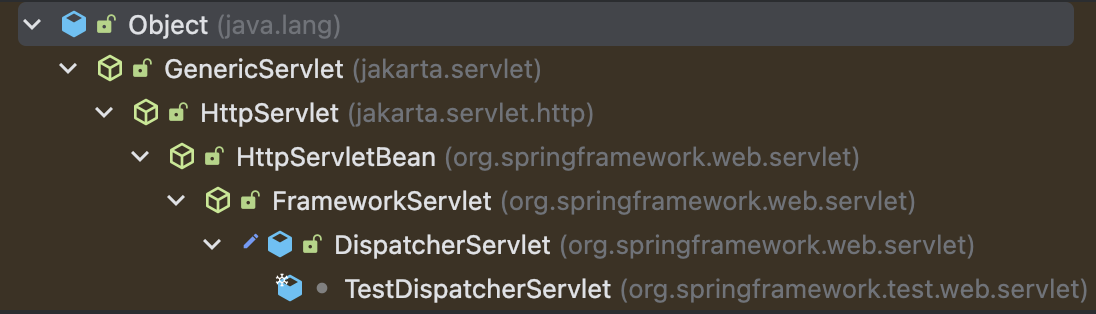
Servlet Bean 초기화
HttpServletBean
은 GenericServelt
의 init
를 재정의하고 있다.
해당 메서드 안에서는 FrameworkServlet
이 재정의한 HttpServletBean
의 initServletBean
을 실행한다.
public final void init() throws ServletException {
// ...
// Let subclasses do whatever initialization they like.
initServletBean();
}
webApplicationContext 초기화
FrameworkServlet
의 initServletBean
에서는 initWebApplicationContext
를 통해 webApplicationContext
가 초기화된다.
// FrameworkServlet
protected final void initServletBean() throws ServletException {
// ...
try {
this.webApplicationContext = initWebApplicationContext();
initFrameworkServlet();
}
catch (ServletException | RuntimeException ex) {
logger.error("Context initialization failed", ex);
throw ex;
}
// ..
}
DispatcherServlet 갱신
이후 webApplicationContext
이 DispatcherServlet
이 재정의한 FrameworkServelet
의 onRefresh
메서드를 통해 스프링에서 설정하여 빈으로 등록된 MultipartResolver
, LocaleResolver
, ThemeResolver
, HandlerMappings
, HandlerAdapters
, HandlerExceptionResolvers
, RequestToViewNameTranslator
, ViewResolvers
, FlashMapManager
가 초기화된다.
// DispatcherServlet
protected WebApplicationContext initWebApplicationContext() {
// ..
if (!this.refreshEventReceived) {
// Either the context is not a ConfigurableApplicationContext with refresh
// support or the context injected at construction time had already been // refreshed -> trigger initial onRefresh manually here. synchronized (this.onRefreshMonitor) {
onRefresh(wac);
}
}
// ..
}
protected void onRefresh(ApplicationContext context) {
initStrategies(context);
}
protected void initStrategies(ApplicationContext context) {
initMultipartResolver(context);
initLocaleResolver(context);
initThemeResolver(context);
initHandlerMappings(context);
initHandlerAdapters(context);
initHandlerExceptionResolvers(context);
initRequestToViewNameTranslator(context);
initViewResolvers(context);
initFlashMapManager(context);
}
요청 처리
위와 같은 과정을 통해 요청을 처리할 준비를 마무리하고 서버가 시작되며 실제 요청을 처리한다.DispatcherServlet
의 doService
를 통해 요청 처리가 이루어지는데 이때 HandlerMethod
를 사용하여 요청을 처리한다.
이 과정에서 HandlerMethod
는 아직 실제 빈이 주입되어 있지 않을 수 있다. 이를 위해 요청을 처리할 땐 createWithResolvedBean
에서 HandlerMethod
에 등록된 빈이 String
타입의 빈 이름이라면 실제 빈으로 교체한 이후 요청을 처리한다.
public HandlerMethod createWithResolvedBean() {
Object handler = this.bean;
if (this.bean instanceof String beanName) {
Assert.state(this.beanFactory != null, "Cannot resolve bean name without BeanFactory");
handler = this.beanFactory.getBean(beanName);
}
Assert.notNull(handler, "No handler instance");
return new HandlerMethod(this, handler, false);
}
'스프링' 카테고리의 다른 글
Webflux, ReactiveTransactionManager 환경에서의 이벤트 발행 (0) | 2025.03.24 |
---|---|
인터페이스 타입으로 등록한 빈을 구현체 타입으로 DI 받지 못한 이유 (0) | 2025.03.19 |
트랜잭션을 위한 프록시 객체와 AspectJ의 프록시 객체는 어떤 순서로 실행될까? (0) | 2025.03.18 |
내가 이해한 스프링의 빈 생명 주기 (0) | 2025.03.17 |
트랜잭션 커밋과 AOP 중 어떤 것이 먼저 실행 될까? (0) | 2025.03.15 |